Handling events in ReactJS
Handling events in any react application is inevitable. ReactJS events are just like javascript events they both just differ in a syntatical way.(i.e the way of writing the code). As we know ReactJS provides an elegant and simple syntax when compare to the raw javascript.
Simple Click Event in ReactJS
- Handling events in react easy very simple.
- Follow camel case notations rather than lowercase.
- Try to use arrow functions wherever possible because they are faster.
- Let’s write some code to explore events in reactjs
Html Code
<div id="root">
</div>
ReactJS Code
class LogoToggle extends React.Component {
constructor(props) {
super(props);
this.state = {
toggle: true,
};
this.handleClick = this.handleClick.bind(this);
}
handleClick() {
this.setState(prevState => ({
toggle: !prevState.toggle
}));
}
render() {
if(this.state.toggle){
return (
<button onClick={this.handleClick}>
{this.state.toggle ? 'Show Agiliq Logo' : 'Hide Agiliq Logo'}
</button>
);
}else{
return(
<div>
<button onClick={this.handleClick}>
{this.state.toggle ? 'Show Agiliq Logo' : 'Hide Agiliq Logo'}
</button>
<img width="300" src="http://books.agiliq.com/projects/django-design-patterns/en/latest/_static/logo.png"/>
</div>
);
}
}
}
ReactDOM.render(
<LogoToggle />,
document.getElementById('root')
);
- you can check it out in codepen for live example
- In above code we have bind the function
handleClick
to the button. Based on thetoggle
variable in the state we are rendering theLogoToggle
component. - If
toggle
is set to “true” then we are hiding the image otherwise we are displaying the image. - In above example we have just used the
click
event. We can also use more events likeonClickCapture
,onKeyDown
,onKeyPress
, etc. - You can checkout these events in react official documentation at https://reactjs.org/docs/events.html
Types of Event Binding in ReactJs
- For every component we have a state. In most of the cases we use it.
- State is not available directly on the component method/function.
- We have to externally bind
this
keyword to the method/function in order to use component state. - We can achieve it in two ways. 1. Binding in the constructor method, 2. Binding in the render method.
Binding in the constructor method
class MyComponent extends Component {
constructor() {
this.handleClick = this.handleClick.bind(this);
}
handleClick(){
// do something
}
render() {
return (<button onClick={this.handleClick}/>);
}
}
- In above code we have overriden the method
component
and binded the state.
Binding in the render method
class MyComponent extends Component {
handleClick(){
// do something
}
render() {
return (<button onClick={this.handleClick.bind(this)}/>);
}
}
- In above code we have dynamically binded the state in the render method.
Points to Note
- The best place to bind the event handlers is within the constructor because if we use the event handler in more than once then it will lead to performance issues.
- Do not bind
this
to event handler method if event handler does not requires component state.
Thank you for reading the Agiliq blog. This article was written by Anjaneyulu Batta on Jan 22, 2019 in javascript , reactjs , handling events .
You can subscribe ⚛ to our blog.
We love building amazing apps for web and mobile for our clients. If you are looking for development help, contact us today ✉.
Would you like to download 10+ free Django and Python books? Get them here
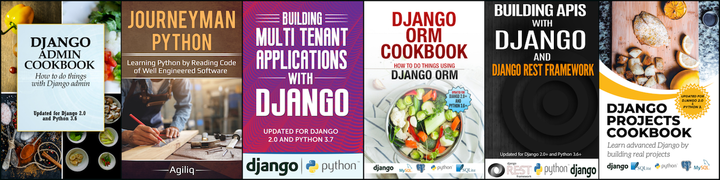