This is not yet another Django vs Rails blog post. It is a compilation of notes I made working with Django after having worked on Rails for years.
In this post I want to give a brief introduction to Django project layout from a Rails developer point of view, on what is there, what is not there and where to look for things. It should help a rails developer working on django be able to find the necessary files and underatnd the layout of the project files.
Once you have both the frameworks installed on your system you can create the projects using the commands
# creating a rails project
rails rails_project
# creating a Django project
django-admin.py startproject django_project
Lets look at the files and structure created by the respective frameworks
#rails_project
README
Rakefile
app/
controllers/
application_controller.rb
helpers/
application_helper.rb
models/
views/
layouts/
config/
boot.rb
database.yml
environment.rb
environments/
development.rb
production.rb
test.rb
initializers/
backtrace_silencers.rb
inflections.rb
mime_types.rb
new_rails_defaults.rb
session_store.rb
locales/
en.yml
routes.rb
db/
seeds.rb
doc/
README_FOR_APP
lib/
tasks/
log/
development.log
production.log
server.log
test.log
public/
404.html
422.html
500.html
favicon.ico
images/
rails.png
index.html
javascripts/
application.js
controls.js
dragdrop.js
effects.js
prototype.js
robots.txt
stylesheets/
script/
about
console
dbconsole
destroy
generate
performance/
benchmarker
profiler
plugin
runner
server
test/
fixtures/
functional/
integration/
performance/
browsing_test.rb
test_helper.rb
unit/
tmp/
cache/
pids/
sessions/
sockets/
vendor/
plugins/
that is huge….
lets look at the django project files
# django_project
__init__.py
manage.py
settings.py
urls.py
far lesser when compared to the rails project.
In fact a rails project comes with everything a web application needs. When I say everything I mean everything….. base application, routing, database configuration, development, test and production environment specific configurations and their respective log files, javascript, test, some standard html files and some helpful scripts for developing.
Why then does a Django project have so less number of files? It has got to do with the Django’s philosophy and the concept of applications. The django project is not complete without the application, so lets create a application inside the project and have a look at the structure
django-admin.py startapp app
# django_project
__init__.py
app/
__init__.py
models.py
tests.py
views.py
manage.py
settings.py
urls.py
even after including this, the number of files is still less than the rails project.
Lets break it down and relate both the frameworks.
Rails | Django | |
---|---|---|
Configuration Files |
|
settings.py, one file for everything, database configuration and any other configuaration or settings will be in this file |
URLs | config/routes.rb | urls.py |
Schema/Models |
|
|
Management Commands |
|
manage.py is the file for all your tasks
|
Application Code | app/controllers/* will contain the application logic | views.py file under each application folder is the place to write to your application logic, file can be named with any name, views.py is the general convention |
Application templates |
app/views/
|
</tr>
</tbody>
</table>
and lets have a look at the other things in rails_project
|
Thank you for reading the Agiliq blog. This article was written by dheeru on Nov 26, 2009 in python .
You can subscribe ⚛ to our blog.
We love building amazing apps for web and mobile for our clients. If you are looking for development help, contact us today ✉.
Would you like to download 10+ free Django and Python books? Get them here
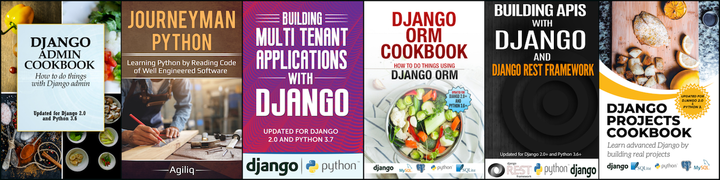